Confronto di stringhe VBA di Excel
Per confrontare due stringhe in VBA abbiamo una funzione incorporata ovvero " StrComp ". Possiamo leggerlo come " Confronto stringhe ", questa funzione è disponibile solo con VBA e non è disponibile come funzione del foglio di lavoro. Confronta due stringhe qualsiasi e restituisce i risultati come "Zero (0)" se entrambe le stringhe corrispondono e se entrambe le stringhe fornite non corrispondono, otterremmo "Uno (1)" come risultato.
In VBA o Excel, affrontiamo molti scenari diversi. Uno di questi scenari è "confrontare due valori di stringa". In un normale foglio di lavoro, possiamo farlo in diversi modi, ma in VBA, come si fa?

Di seguito è riportata la sintassi della funzione "StrComp".

Innanzitutto, due argomenti sono abbastanza semplici,
- per String 1, dobbiamo fornire quale sia il primo valore che stiamo confrontando e
- per la stringa 2, dobbiamo fornire il secondo valore che stiamo confrontando.
- (Confronta) questo è l'argomento opzionale della funzione StrComp. Questo è utile quando vogliamo confrontare il confronto con distinzione tra maiuscole e minuscole. Ad esempio, in questo argomento, "Excel" non è uguale a "EXCEL" perché entrambe queste parole fanno distinzione tra maiuscole e minuscole.
Possiamo fornire tre valori qui.
- Zero (0) per " Confronto binario " , ovvero "Excel", non è uguale a "EXCEL". Per il confronto case sensitive, possiamo fornire 0.
- Uno (1) per " Confronta testo " , ovvero "Excel", è uguale a "EXCEL". Questo è un confronto senza distinzione tra maiuscole e minuscole.
- Due (2) solo per il confronto del database.
I risultati della funzione "StrComp" non sono VERO o FALSO per impostazione predefinita, ma variano. Di seguito sono riportati i diversi risultati della funzione "StrComp".
- Otterremo "0" come risultato se le stringhe fornite corrispondono.
- Otterremo "1" se le stringhe fornite non corrispondono e, in caso di corrispondenza numerica, otterremo 1 se la stringa 1 è maggiore della stringa 2.
- Otterremo "-1" se il numero della stringa 1 è inferiore al numero della stringa 2.
Come eseguire il confronto delle stringhe in VBA?
Esempio 1
Assoceremo " Bangalore " alla stringa " BANGALORE ".
Innanzitutto, dichiara due variabili VBA come stringa per memorizzare due valori di stringa.
Codice:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String End Sub

Per queste due variabili, memorizzare due valori di stringa.
Codice:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" End Sub
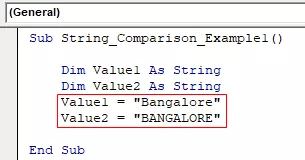
Dichiarare ora un'altra variabile per memorizzare il risultato della funzione " StrComp ".
Codice:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String End Sub

Per questa variabile, aprire la funzione "StrComp".
Codice:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (End Sub
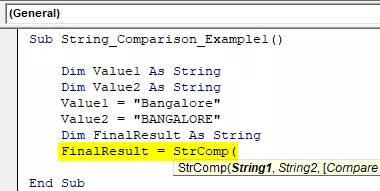
Per "String1" e "String2" abbiamo già assegnato valori tramite variabili, quindi inserisci i nomi delle variabili, rispettivamente.
Codice:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, End Sub
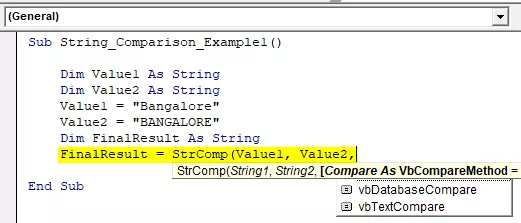
L'ultima parte della funzione è "Confronta" per questa scelta "vbTextCompare".
Codice:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, vbTextCompare) End Sub

Ora mostra la variabile "Risultato finale" nella finestra di messaggio in VBA.
Codice:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, vbTextCompare) MsgBox FinalResult End Sub

Ok, eseguiamo il codice e vediamo il risultato.
Produzione:

Poiché entrambe le stringhe "Bangalore" e "BANGALORE" sono uguali, abbiamo ottenuto il risultato come 0, ovvero corrispondenza. Entrambi i valori fanno distinzione tra maiuscole e minuscole poiché abbiamo fornito l'argomento come "vbTextCompare" , ha ignorato la corrispondenza con distinzione tra maiuscole e minuscole e ha fatto corrispondere solo i valori, quindi entrambi i valori sono gli stessi e il risultato è 0, ovvero TRUE.
Codice:
Sub String_Comparison_Example1 () Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp (Value1, Value2, vbTextCompare) MsgBox FinalResult End Sub

Esempio n. 2
For the same code, we will change the compare method from “vbTextCompare” to “vbBinaryCompare.”
Code:
Sub String_Comparison_Example2() Dim Value1 As String Dim Value2 As String Value1 = "Bangalore" Value2 = "BANGALORE" Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Now run the code and see the result.
Output:

Even though both the strings are the same, we got the result as 1, i.e., Not Matching because we have applied the compare method as “vbBinaryCompare,” which compares two values as case sensitive.
Example #3
Now we will see how to compare numerical values. For the same code, we will assign different values.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 500 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Both the values are 500, and we will get 0 as a result because both the values are matched.
Output:
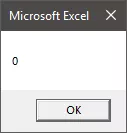
Now I will change the Value1 number from 500 to 100.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 1000 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Run the code and see the result.
Output:
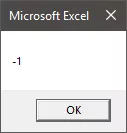
We know Value1 & Value2 aren’t the same, but the result is -1 instead of 1 because for numerical comparison when the String 1 value is greater than String 2, we will get this -1.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 1000 Value2 = 500 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Now I will reverse the values.
Code:
Sub String_Comparison_Example3() Dim Value1 As String Dim Value2 As String Value1 = 500 Value2 = 1000 Dim FinalResult As String FinalResult = StrComp(Value1, Value2, vbBinaryCompare) MsgBox FinalResult End Sub

Run the code and see the result.
Output:

This is not special. If not match, we will get 1 only.
Things to Remember here
- (Compare) argument of “StrComp” is optional, but in case of case sensitive match, we can utilize this, and the option is “vbBinaryCompare.”
- The result of numerical values is slightly different in case String 1 is greater than string 2, and the result will be -1.
- Results are 0 if matched and 1 if not matched.
Recommended Articles
Questa è stata una guida al confronto delle stringhe VBA. Qui discutiamo come confrontare due valori di stringa utilizzando la funzione StrComp in Excel VBA insieme a esempi e scaricare un modello Excel. Puoi anche dare un'occhiata ad altri articoli relativi a Excel VBA -
- Guida alle funzioni stringa VBA
- VBA Split String in Array
- Metodi di sottostringa VBA
- Testo VBA